Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial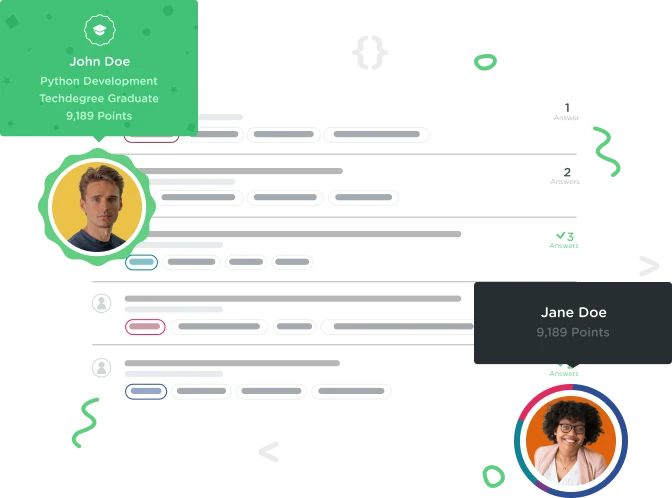

Dax Allen
4,788 PointsBank Account challenge problem.
Implement a to_s method on the BankAccount class that displays the name and balance in the following format (without brackets): Name: [name], Balance: [balance] It is not necessary to use the sprintf method to implement the balance printing.
I keep getting the error: The 'to_s' method did not return a correctly formatted string.
I have no idea what I am doing wrong. Any help is appreciated.
class BankAccount
attr_reader :name
def initialize(name)
@name = name
@transactions = []
add_transaction("Beginning Balance", 0)
end
def balance
balance = 0
@transactions.each do |transaction|
balance += transaction[:amount]
end
balance
end
def debit(description, amount)
add_transaction(description, -amount)
end
def credit(description, amount)
add_transaction(description, amount)
end
def add_transaction(description, amount)
@transactions.push(description: description, amount: amount)
end
def to_s
puts "Name: #{name}, Balance: #{balance}"
end
end
3 Answers
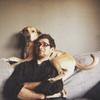
Joshua Shroy
9,943 PointsHey Dax,
Instead of puts
which displays the string , try return
- which returns the value.
def to_s
return "Name: #{name}, Balance: #{balance}"
end
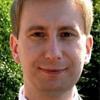
Tomasz Necek
Courses Plus Student 7,028 PointsWhy this one is wrong ?
"The to_s
method did not return a correctly formatted string."
def to_s
puts "Name: #{@name}, " + "Balance: " + sprintf("%0.2f", balance)
end
??:)

Nate Heinold
2,679 PointsI was interested in this as well. Thinking is that, unlike the training video, our code challenge does not have the trailing calls on the class:
...
puts "Register:"
bank_account.print_register
So to display "Name: #{name}, Balance: #{balance}" by simply running the Class (as the code challenge exists), you need to use return instead of puts .

Anterrio Howard
2,625 PointsHere is what it should be.
class BankAccount attr_reader :name
def initialize(name) @name = name @transactions = [] add_transaction("Beginning Balance", 0) end
def balance balance = 0 @transactions.each do |transaction| balance += transaction[:amount] end balance end
def debit(description, amount) add_transaction(description, -amount) end
def credit(description, amount) add_transaction(description, amount) end
def add_transaction(description, amount) @transactions.push(description: description, amount: amount) end
def to_s return "Name: #{name}, Balance: #{balance}" end end
Dax Allen
4,788 PointsDax Allen
4,788 PointsThat worked. Thank you for your help.
Joshua Shroy
9,943 PointsJoshua Shroy
9,943 PointsNo problem!