Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial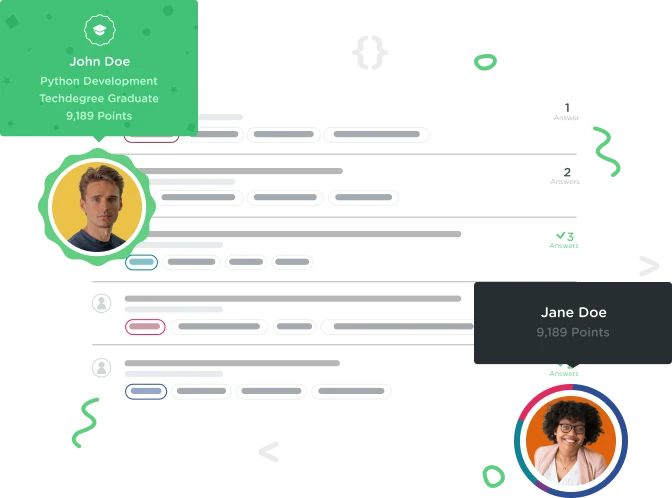

Brandon Pence
1,089 PointsConfused about why location.X and location.Y is used to call the coordinates.
if "location" is an instance of the MapLocation class, and MapLocation class is a subclass of the Point class, why does the reference to location's variables use lower case x and y?
The Point class has an upper case X and Y, which would make sense to me if someone referenced those variables in an object of that class, but why would an instance of MapLocation use upper case X and Y when the MapLocation sub-class uses int x, int y and base (x, y) in its constructor?
I guess I'm just confused on the logic of how these variables are being inherited and referenced. I think the similarity of variables (lower case vs upper case) may be obscuring the meaning of the syntax for me in this case.
I would have expected that a reference to MapLocation location's X and Y coordinates would instead say (location.x + "," + location.y). I commented above the specific code in question. I thought I had the concept of inheritance down after a couple reviews of the lesson, but it appears I'm still struggling.
Thanks! Brandon
//Point Class
using System;
namespace TreehouseDefense
{
class Point
{
public readonly int X;
public readonly int Y;
public Point(int x, int y)
{
X = x;
Y = y;
}
public int DistanceTo(int x, int y)
{
return (int)Math.Sqrt(Math.Pow(X-x, 2) + Math.Pow(Y-y, 2));
}
}
}
//MapLocation class
namespace TreehouseDefense
{
class MapLocation : Point
{
public MapLocation(int x, int y, Map map) : base (x, y)
{
if (!map.OnMap(this))
{
throw new System.Exception(x + ", " + y + " is outside the boundaries of the map");
}
}
}
}
//Main method that references location.X and location.Y
namespace TreehouseDefense
{
class Game
{
public static void Main()
{
Map map = new Map(8, 5);
try
{
Path path = new Path(
new [] {
new MapLocation(0, 2, map),
new MapLocation(1, 2, map),
new MapLocation(2, 2, map),
new MapLocation(3, 2, map),
new MapLocation(4, 2, map),
new MapLocation(5, 2, map),
new MapLocation(6, 2, map),
new MapLocation(7, 2, map),
new MapLocation(8, 2, map),
}
);
MapLocation location = path.GetLocationAt(8);
//code in question
if(location != null)
{
Console.WriteLine(location.X + "," + location.Y);
}
}
catch(OutOfBoundsException ex)
{
Console.WriteLine(ex.Message);
}
catch(TreehouseDefenseException ex)
{
Console.WriteLine("Unhandled TreehouseDefenseException");
}
catch(Exception ex)
{
Console.WriteLine("Unhandled Exception: " + ex);
}
}
}
}
1 Answer
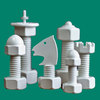
Steven Parker
240,995 PointsThe references use upper case X
and Y
, because those are the variables inside the Point class.
The lower case "x" and "y" are only parameters of the constructors (for both Point and MapLocation), and they are copied into the permanent variables in the constructor of the Point class.
It might be a bit more clear if you rename the lower case "x" and "y" to something like "argx" and "argy" respectively, to help distinguish them from the class variables.