Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial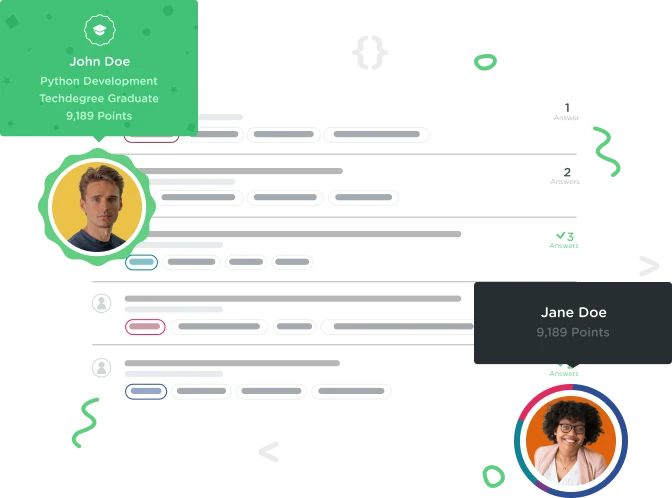

Michael North
2,561 PointsI can't understand why not working
In my code I can't understand why its not working. I think I'm a little stuck on static members, not fully sure what they are. Any help is appreciated :).
using System;
namespace Treehouse.CodeChallenges
{
class RightTriangle
{
static double Hypotenuse(double side1, double side2) = new Hypotenuse()
{
return Math.Sqrt(side1 * side1 + side2 * side2);
}
}
}
3 Answers

andren
28,558 PointsStatic members aren't really any different from normal members as far as declaration goes besides having to use the static
keyword. The difference behavior wise is that the member can be accesses directly from the class, without having to instantiate the class and call the method on that instantiated version. Which is what you normally have to do.
There are three issues with your code:
The method is meant to be called CalculateHypotenuse not simply Hypotenuse.
= new Hypotenuse()
does not belong at the end of the method declaration, as mentioned static methods aren't declared any differently from normal methods, besides the use of thestatic
keyword.The method needs to be
public
, since it's intended to be called outside of the class.
If you fix those issues like this:
using System;
namespace Treehouse.CodeChallenges
{
class RightTriangle
{
public static double CalculateHypotenuse (double side1, double side2)
{
return Math.Sqrt(side1 * side1 + side2 * side2);
}
}
}
Then your code will work.

Krishna Pratap Chouhan
15,203 Pointsits
return System.Math.Sqrt()
System is the namespace you should include.

andren
28,558 PointsSince he includes the line using System;
at the top of the file he does not need to specify System
when calling Math
within this file. That's the main point of the using
keyword, it allows you to use something from a namespace without having to constantly reference that namespace over and over again within the code.

Michael North
2,561 Pointsthank you