Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial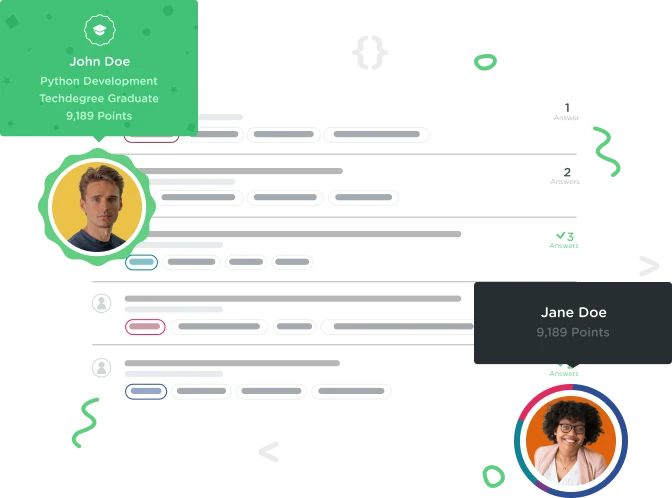

yebeen Lee
4,855 Pointsindex.js
var http = require("http"); var routes = require("./routes.js");
http.createServer(function(request, response) { if (request.url === "/") { routes.root(request, response); // Calls root() method for '/' } else if (request.url === "/contact") { routes.contact(request, response); // Calls contact() method for '/contact' } else if (request.url === "/about") { routes.about(request, response); // Calls about() method for '/about' } else { // If no route matches, return a 404 response response.writeHead(404, {'Content-type': "text/plain"}); response.end("Not Found\n"); } }).listen(3000, function() { console.log("Server is running on port 3000"); });
function root(request, response) {
if(request.url === "/") {
response.writeHead(200, {'Content-type': "text/plain"});
response.end("Home\n");
}
}
function contact(request, response) {
if(request.url === "/contact") {
response.writeHead(200, {'Content-type': "text/plain"});
response.end("Contact\n");
}
}
function about(request, response) {
if(request.url === "/about") {
response.writeHead(200, {'Content-type': "text/plain"});
response.end("About\n");
}
}
// Exporting all the functions
module.exports = {
root: root,
contact: contact,
about: about
};
var http = require("http");
var routes = require("./routes.js");
http.createServer(function(request, response) {
if (request.url === "/") {
routes.root(request, response); // Calls root() method for '/'
} else if (request.url === "/contact") {
routes.contact(request, response); // Calls contact() method for '/contact'
} else if (request.url === "/about") {
routes.about(request, response); // Calls about() method for '/about'
} else {
// If no route matches, return a 404 response
response.writeHead(404, {'Content-type': "text/plain"});
response.end("Not Found\n");
}
}).listen(3000, function() {
console.log("Server is running on port 3000");
});
1 Answer
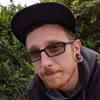
Travis Alstrand
Treehouse Project ReviewerI didn't see any question here, but I went ahead and put your code into the challenge. The first one passed and the second one failed. In index.js
, I undid back to what was originally there and simply added a line for the about method similar to the other two before it. We don't need to add everything else in there that you have currently. These challenges can be very picky about what they want so we need to read the challenge closely and try not to add in extra unnecessary things 💪
var http = require("http");
var routes = require("./routes.js");
http.createServer(function(request, response){
routes.root(request, response);
routes.contact(request, response);
routes.about(request, response);
}).listen(3000);
In the future, please provide some context into the issue that you're having whether it be an error message, or what you don't understand about the challenge, etc. You can also take a look at this video about Posting a Question. 👍