Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial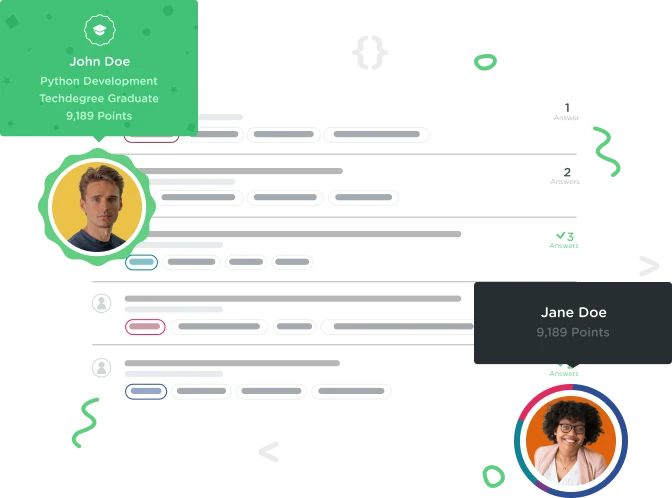
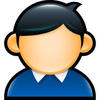
Daniel Hildreth
16,170 PointsMy SoccerStats code does not compile and build the same way.
My code does not compile the same way as hers does in the video. It looks exactly the same. When I CTRL + F5 to run it, I do not get the double lines that she is getting. I then downloaded the project files and ran that code, and it broke it into double lines. I then copied the code into my project, but I still do not get double lines. Could someone tell me if there is something wrong with my code or my IDE?
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace SoccerStats
{
class Program
{
static void Main(string[] args)
{
string currentDirectory = Directory.GetCurrentDirectory();
DirectoryInfo directory = new DirectoryInfo(currentDirectory);
var fileName = Path.Combine(directory.FullName, "SoccerGameResults.csv");
var fileContents = ReadFile(fileName);
string[] fileLines = fileContents.Split(new char[] { '\r', '\n' });
foreach (var line in fileLines)
{
Console.WriteLine(line);
}
}
public static string ReadFile(string fileName)
{
using (var reader = new StreamReader(fileName))
{
return reader.ReadToEnd();
}
}
}
}
6 Answers
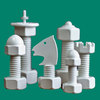
Steven Parker
240,995 PointsAre you using a Mac or Linux?
I notice the lines are being split using both carriage return ('\r'
) and line feed ('\n'
). In Windows, both of these are present at the end of the line, which will produce an extra blank line between the content lines.
But on Mac or Linux, the line feed is used alone as the end of line, so the split would not generate the blank lines.
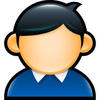
Daniel Hildreth
16,170 PointsI am using Windows 8 with Visual Studio 2015.

Erick R
5,293 PointsCame here to agree with all of you. It seems that my compiler ignores \r and only acknowledges \n as a carriage return when used in conjunction.

William Schultz
2,926 PointsI'm guessing the IDE or something was updated to only use \n because I am using Windows 7 with VS 2015 and I only get \n as well. I had no reason to follow the whole StringSplitOptions part of the video.
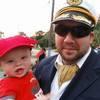
Christian Higgins
15,758 PointsSeeing this problem too. No double line split on Windows 10, Visual Studio 2017

Thomas Heaney
2,423 PointsIssue persists with Windows 10, VS 2019.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace SoccerStats
{
class Program
{
static void Main(string[] args)
{
string currentDirectory = Directory.GetCurrentDirectory();
DirectoryInfo directory = new DirectoryInfo(currentDirectory);
var fileName = Path.Combine(directory.FullName, "SoccerGameResults.csv");
var fileContents = ReadFile(fileName);
string[] fileLines = fileContents.Split(new char[] { '\r', '\n' });
foreach(var line in fileLines)
{
Console.WriteLine(line);
}
}
public static string ReadFile(string fileName)
{
using(var reader = new StreamReader(fileName))
{
return reader.ReadToEnd();
}
}
}
}