Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial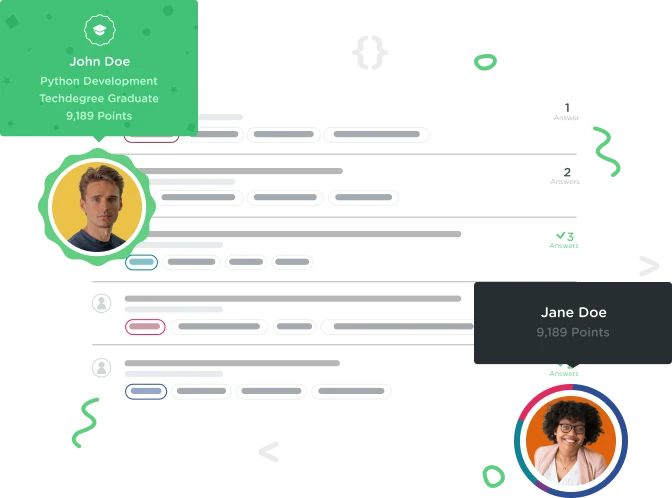

Steven Scott
7,542 PointsNot sure what I am doing wrong here. Cans someone help me answer this question and provide a working answer.
In IssuesController.cs add another action method named Report to handle HTTP posts from the form in the Report.cshtml view.
Decorate the action method with an attribute so that MVC will only route POST requests to it. Add a total of six parameters that match the form field names in the Report.cshtml view file in order to capture posted values from the form. Remember to change the first letter of each form field to lowercase (i.e. "Name" to "name"). Use the string data type for each parameter. For now, in the action method, just return a call to the View method.
using System.Web.Mvc;
namespace IssueReporter.Controllers
{
public class IssuesController : Controller
{
public ActionResult Report()
{
return View();
}
[report("Report")]
[HttpPost]
public ActionResult Report(
string name, string email, string departmentId, string severity, string reproducible, string descriptionOfProblem)
{
return View();
}
}
Report.cshtml
@{
ViewBag.Title = "Report an Issue";
}
<h2>@ViewBag.Title</h2>
<form method="post">
<div>
<label for="Name">Name</label>
<input type="text" id="Name" name="Name" />
</div>
<div>
<label for="Email">Email</label>
<input type="text" id="Email" name="Email" />
</div>
<div>
<label for="DepartmentId">Department</label>
<input type="text" id="DepartmentId" name="DepartmentId" />
</div>
<div>
<label for="Severity">Severity</label>
<input type="text" id="Severity" name="Severity" />
</div>
<div>
<label for="Reproducible">Reproducible</label>
<input type="text" id="Reproducible" name="Reproducible" />
</div>
<div>
<label for="DescriptionOfProblem">Description of Problem</label>
<textarea id="DescriptionOfProblem" name="DescriptionOfProblem"></textarea>
</div>
<button type="submit">Save</button>
</form>
3 Answers
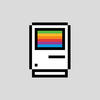
Hakim Rachidi
38,491 PointsSorry, I forgot to say that you have to leave the predefined controller.
In the end Issuecontroller.cs should look something like this:
using System.Web.Mvc;
namespace IssueReporter.Controllers
{
public class IssuesController : Controller
{
public ActionResult Report()
{
return View();
}
[HttpPost]
public ActionResult Report(
string name, string email, string departmentId, string severity, string reproducible, string descriptionOfProblem)
{
return View();
}
}
}
Now you can just copy it and paste it in to the challenge ;) But don't make any changes to the view.
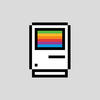
Hakim Rachidi
38,491 PointsI wonder why you added a report Attribute (in the Controller).
[report("Report")]
All in all just leave it out and everything should work.

Steven Scott
7,542 PointsDidn't realize i did that. But I just took it out and it still doesn't work.
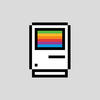
Hakim Rachidi
38,491 PointsTry out the following steps:
- Copy your controller out of your question
- Reset the challenge and exchange the predefined controller with yours (clipboard)
- Delete the Report Attribute
In my case this works fine.

Steven Scott
7,542 Pointsusing System.Web.Mvc;
namespace IssueReporter.Controllers { public class IssuesController : Controller { public ActionResult Report() { return View(); } HttpPost] public ActionResult Report(
string name, string email, string departmentId, string severity, string reproducible, string descriptionOfProblem)
{
return View();
}
}
}

Steven Scott
7,542 PointsTook out the [report("Report")] .
tried copy and paste it from the clipboard and restart it. still didn't work
Hakim Rachidi
38,491 PointsHakim Rachidi
38,491 PointsTake a look
In the comment above you forgot the opening bracket for the Attribute:
This could be the mistake.
Steven Scott
7,542 PointsSteven Scott
7,542 PointsHmm that worked I think the problem was it was actually missing a closing curly brace at the end. Noticed that after comparing the 2 codes side by side.
Thanks alot for the help always good to have a second set of eyes or a working code so you can see were you missed something even if it is just a closing tag or a typo.