Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial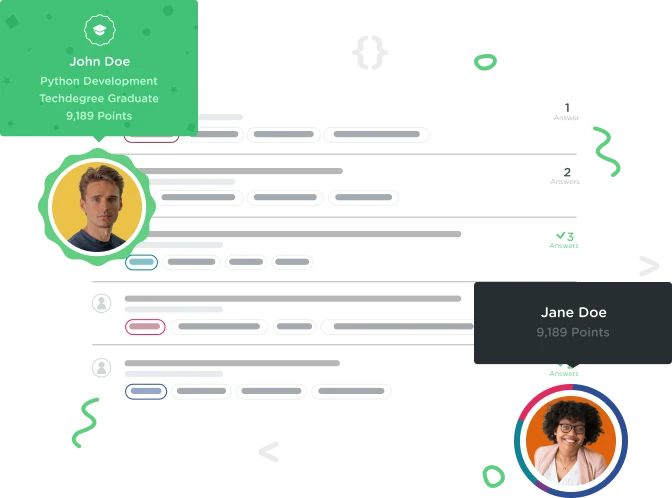
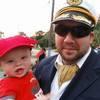
Christian Higgins
15,758 PointsProgram.cs(70,8): error CS1525: Unexpected symbol `}'
Not sure why this bracket is extra.
using System;
using System.IO;
using System.Collections.Generic;
using Newtonsoft.Json;
namespace Treehouse.CodeChallenges
{
public class Program
{
public static void Main(string[] arg)
{
}
public static WeatherForecast ParseWeatherForecast(string[] values)
{
var weatherForecast = new WeatherForecast();
weatherForecast.WeatherStationId = values[0];
DateTime timeOfDay;
if (DateTime.TryParse(values[1], out timeOfDay))
{
weatherForecast.TimeOfDay = timeOfDay;
}
Condition condition;
if (Enum.TryParse(values[2], out condition))
{
weatherForecast.Condition = condition;
}
int temperature;
if (int.TryParse(values[3], out temperature))
{
weatherForecast.Temperature = temperature;
}
double precipitation;
if (double.TryParse(values[4], out precipitation))
{
weatherForecast.PrecipitationChance = precipitation;
}
if (double.TryParse(values[5], out precipitation))
{
weatherForecast.PrecipitationAmount = precipitation;
}
return weatherForecast;
}
public static List<WeatherForecast> DeserializeWeather(string fileName)
{
var weatherForecasts = new List<WeatherForecast>();
using (var reader = new StreamReader(fileName))
using (var jsonReader = new JsonTextReader(reader))
{
var serializer = new JsonSerializer();
weatherForecasts = serializer.Deserialize<List<WeatherForecast>>(jsonReader);
}
return weatherForecasts;
}
/*
* Inside the SerializeWeatherForecast Instantiate a new JsonSerializer
* and name it serializer.
* Then, add a using statement wrapped around a
* new StreamWriter named writer. Pass the StreamWriter constructor the
* fileName parameter.
*/
public static void SerializeWeatherForecasts(List<WeatherForecast> weatherForecasts, string fileName)
{
var serializer = new JsonSerializer();
using (var writer = new StreamWriter(fileName))
} /* <- This is Line 70 where the compile error is */
}
}
2 Answers
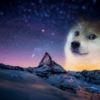
Connor Walker
17,985 PointsIn C# a using statement is expected to have some form of scope after it, in the same way that an if statement or loop would have. In your code you haven't provided it any scope so it's not expecting to see the closing curly braces.
Like with if statements and loops the compiler will allow you to have a single statement inside that scope without the need for curly braces, like this:
// This is valid C#
if (true)
Console.WriteLine("This statement is true");
// This is also valid C#
for (int i = 0; i < 10; i++)
if (i % 2 == 0)
Console.WriteLine("Number is even");
When you add a semi colon to the end of the using statement you are giving it a single command, an empty one, but still a command. That makes is valid syntax because of the rule I've shown above.
If you look at the example in the video (I've only skimmed it so but I think this is what you're looking at) you'll see that she has another using statement under the one you've shown.
// This is the same as ...
using (var writer = new StreamWriter(fileName))
using (var jsonWriter = new JsonTextWriter(writer))
{
// Some code here ...
}
// ... this
using (var writer = new StreamWriter(fileName))
{
using (var jsonWriter = new JsonTextWriter(writer))
{
// Some code here ...
}
}
C# ignores white space so that even though it looks like the second using statement is inside the main function scope, it actually belongs to the scope of the using statement above it. Like the if inside the for in my examples.
I haven't used C# in a while so i don't know the answer to the challenge but I hope this helps you understand the problem.
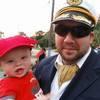
Christian Higgins
15,758 PointsOk, when I added a semicolon after
using (var writer = new StreamWriter(fileName))
it took away the compile error, but comparing it with the code from the videos, we're not supposed to use a semicolon, so.... I'm confused.
Christian Higgins
15,758 PointsChristian Higgins
15,758 PointsIt does help me understand, yes, but in her example, there weren't any brackets under the first using statement, only after the second. That's part of the confusion.
Your example above "This is the same as .... ...this"
That's the confusion.