Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial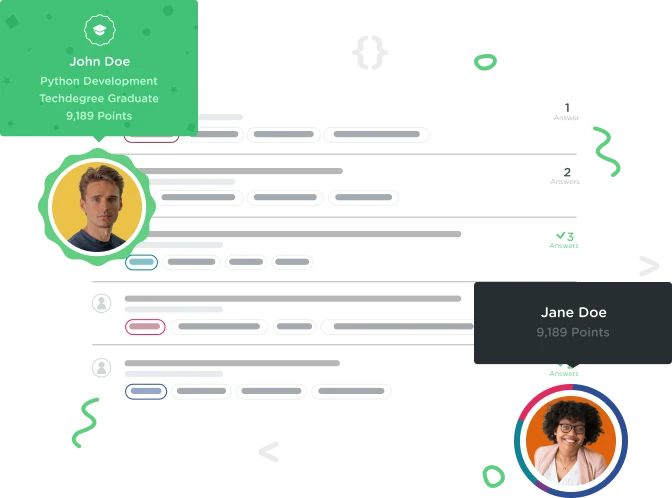

Riley Egan
7,255 Pointstype error help
I'm getting the error "no implicit conversion of String into Integer".
I've been trying a few different things and can't seem to figure out where I'm going wrong. Any help is appreciated!
contact_list = [
{"name" => "Jason", "phone_number" => "123"},
{"name" => "Nick", "phone_number" => "456"}
]
contact_list.each do |item|
puts "Name: #{contact_list["name"]}"
phone_number = contact_list["phone_numnber"]
puts phone_number
end
2 Answers
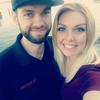
Bryan Reed
11,747 PointsIn your each method your defining 'item' as the Hash in the current iteration, yet in your loop block you're still using contact_list as if it was the Hash and not the Array.
The challenge is also asking to assign the currently iterated hash into a contact variable then printing out each key (name, phone_number)
See below:
contact_list = [
{"name" => "Jason", "phone_number" => "123"},
{"name" => "Nick", "phone_number" => "456"}
]
contact_list.each do |item|
contact = item
puts contact['name']
puts contact['phone_number']
end
William Li
Courses Plus Student 26,868 PointsHi Bryan, thanks for correctly answering the question here. But I'd like to bring your attention to this line contact = item
, you really don't need it for the code, if you want the block parameter to be contact, just name it contact
contact_list.each do |contact|
puts contact['name']
puts contact['phone_number']
end
You can give the block parameter any name, it doesn't have to be item
. And your code becomes little more efficient by getting rid of line contact = item
, as the program now doesn't need to reassign new value to variable contact
at each iteration of the block.
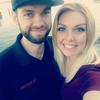
Bryan Reed
11,747 PointsWilliam, I'm glad you pointed that out because it is helpful to know that you can name block parameters whatever you like. I actually debated posting the exact same code you did XD.
The only reason I added the contact = item line is because the challenge explicitly said to do so by stating "Assign each array item to the local variable contact in the block".
William Li
Courses Plus Student 26,868 PointsHi Bryan, :) the code challenge instruction is a little ambiguous here, given how the instructor wrote the Ruby block in the previous video lecture, I believe it actually wants you to name the block parameter contact
. Another thing I'd like to point out is that, block parameter, (sometimes it was referred to as block variable, or block-local variable) is always scoped local to the block, there isn't much distinction between a block parameter VS a local variable created within the block body.
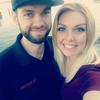
Bryan Reed
11,747 PointsThat makes sense, I'll concede to you there. I didn't go back and watch the previous video. I think what confused me was the word assign in the directions.

Riley Egan
7,255 PointsThank you!
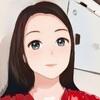
cloud712
7,616 PointsBryan Reed Riley Egan Question on the syntax, what is the difference (if at all) with puts contact['phone_number'] versus puts "#{"contact"["phone_number"]}" ?
I used the second version in my code but didn't realize that the first was an option. It does look cleaner and easier to read.
Riley Egan
7,255 PointsRiley Egan
7,255 PointsHere's the prompt...
Using the each method, iterate over the contact_list array. Assign each array item to the local variable contact in the block and print out the value of the name and phone_number keys.