Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial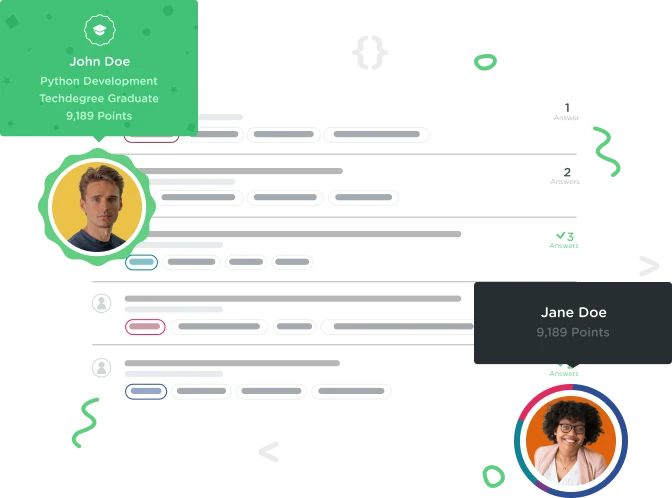

Andrew Walters
8,876 PointsWhat are the benefits to Iterations with Each compared to (while) loops?
Just curious, if (in the example) using a while loop and using iterations with each gives us the same result, why is it beneficial to use one over the other? Could you give me any real world application examples? Thanks
1 Answer
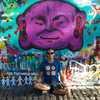
Andrew Stelmach
12,583 PointsYou can use while
and each
iterations for the same thing, but there are things you can do with while
that you can't do with each
. So you can use either thing to, for example, iterate over every element in an array.
each
will always 'do something' for every single element of whatever you are iterating over, UNLESS you use the handy break
method:
array = ["Andy", "Bob", "Chris", "Paul"]
answer = ""
array.each do |name|
answer = gets.chomp
break if answer == name
end
So, we do have a way to halt an each
iteration.
while
will break out and cease an iteration when a certain condition is met by default:
array = ["Andy", "Bob", "Chris", "Paul"]
answer = ""
i = -1
while array[i] != answer do
i += 1
answer = gets.chomp
end
However, you could say that the above is not as clean nor readable as the first example.
However, you couldn't do something like the following with each
:
name = ""
while name != "Andy" do
name = gets.chomp
end
Nor:
array = []
while array.length < 5 do
puts "Enter an item"
item = gets.chomp
array << item
end
Using until
is probably more readable though in this case:
array = []
until array.length == 5 do
puts "Enter an item"
item = gets.chomp
array << item
end
Cheers! Writing this made me realise a couple of things too.
Andrew Walters
8,876 PointsAndrew Walters
8,876 PointsThanks for the info! I thought it might've had to do with the neatness of the code, I just wasn't sure if there were any hidden benefits. I appreciate the help and I'm glad writing to me helped you out too! Cheers :)